Visual Regression Testing: Integrating Comparizen with Playwright
Step-by-step guide to adding visual regression testing to your Playwright projects.
Published on:
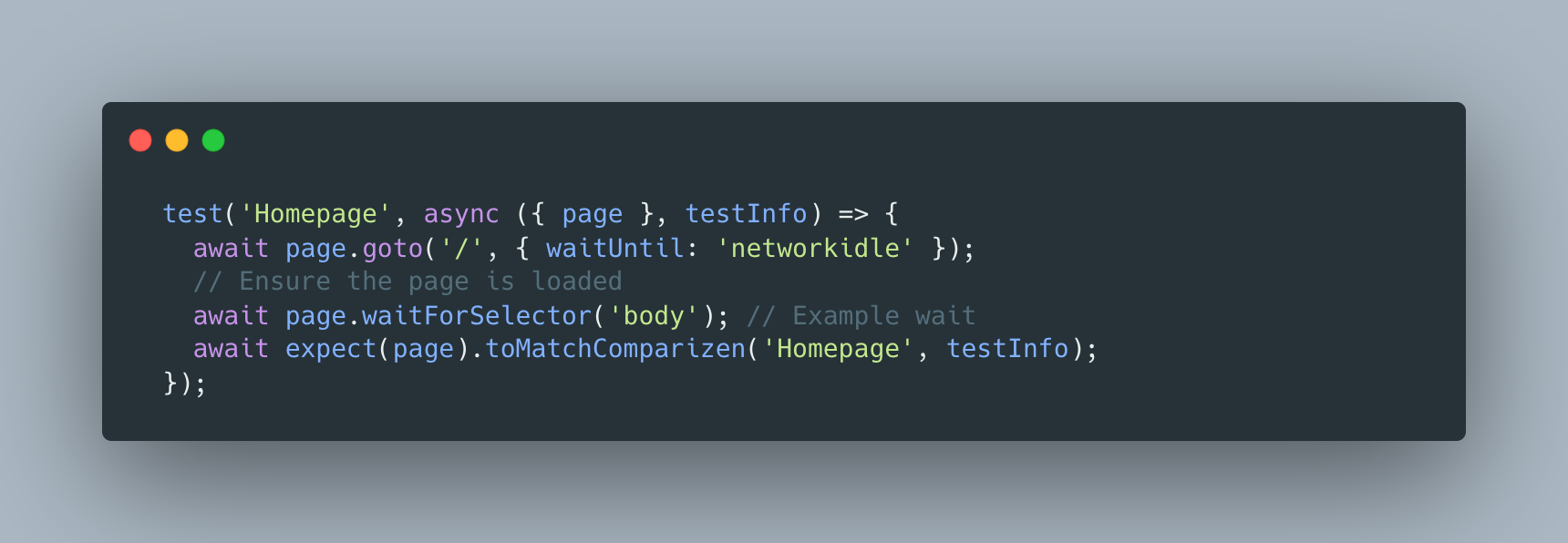
Enhance your Playwright test suite with automated visual regression testing using the comparizen-playwright
package. This step-by-step guide, based on the official Comparizen documentation, shows you how to integrate powerful visual validation into your existing Playwright projects, ensuring UI consistency and catching visual bugs early.
Prerequisites for Comparizen Playwright Integration
Before integrating Comparizen, make sure you have:
- Playwright Installed and Configured: This guide assumes you already have a working Playwright project set up. If not, please follow the official Playwright installation guide first.
- ES Module Support Enabled: Ensure your Playwright project’s
package.json
includes the following line:"type": "module",
Step 1: Install comparizen-playwright Package
Install the necessary comparizen-playwright
package into your Playwright project:
npm install comparizen-playwright
Step 2: Configure Playwright for Comparizen Visual Tests
Modify your playwright.config.ts
file to enable Comparizen visual regression testing:
- Import necessary functions: Add imports for
defineConfig
,expect
from Playwright, andsetupComparizen
fromcomparizen-playwright
. - Call
setupComparizen
: Configure the integration with your Comparizen API key and Project ID. - Add
globalSetup
: Point theglobalSetup
option indefineConfig
to the Comparizen setup script.
Here’s how your playwright.config.ts
might look:
import { defineConfig, expect } from '@playwright/test';
import { setupComparizen } from 'comparizen-playwright';
// Setup Comparizen integration
setupComparizen(expect, {
// make sure to set your own Comparizen api-key and -project-id here.
apiKey: 'your api key',
projectId: 'your project id'
});
export default defineConfig({
// Your existing Playwright config options...
// Add this globalSetup entry
globalSetup: 'node_modules/comparizen-playwright/comparizen-global-setup.js',
// Other config options...
});
Security Note: For optimal security, avoid committing your Comparizen API key directly. Utilize environment variables or tools like dotenv
for secure secret management in your Playwright project.
Step 3: Verify tsconfig.json for Playwright Integration
Ensure a tsconfig.json
file exists at the root of your Playwright project. An empty file suffices if one isn’t present. This step is crucial for TypeScript environments to correctly recognize Comparizen’s extensions to the Playwright expect
assertion library.
Step 4: Implement toMatchComparizen
Assertions in Tests
Integrate visual checks into your Playwright test scripts using the toMatchComparizen
assertion. This powerful command performs the automated visual regression testing comparison against your established baseline.
import { test, expect } from '@playwright/test';
test('Check homepage visuals', async ({ page }, testInfo) => {
await page.goto('https://your-website.com/');
// Perform automated visual comparison with Comparizen
// 'Homepage Visuals' is the unique name for this visual snapshot
await expect(page).toMatchComparizen('Homepage Visuals', testInfo);
// Continue with other standard Playwright functional assertions
await expect(page.locator('h1')).toHaveText('Welcome!');
});
Step 5: Execute Tests and Manage Visual Baselines
Running your tests and managing the visual baseline is a core part of the visual regression testing workflow with Comparizen and Playwright:
-
First Execution: When you run a Playwright test containing a
toMatchComparizen
assertion for the first time with a specific snapshot name, the test will intentionally fail. This occurs because Comparizen needs an initial baseline image for comparison. -
Baseline Review & Approval: Check the Playwright test output log for a link to the Comparizen web application (
https://app.comparizen.com/#/...
). Access this link to review the newly captured snapshot. If the visual appearance is correct,approve
it within Comparizen. This action establishes the snapshot as the official baseline for that specific name. -
Subsequent Test Runs: Execute your Playwright test suite again (e.g.,
npm run test
). Comparizen will now capture new snapshots and perform automated visual comparisons against the approved baseline images. Tests pass if the new snapshots match the baseline (within configured thresholds). If visual differences are detected, the test fails, prompting a review of the comparison details in the Comparizen dashboard.
By following these integration steps, you effectively incorporate automated visual regression testing into your Playwright development cycle. This proactive approach helps catch unintended UI changes, maintain visual consistency across releases, and improve the overall quality of your web application.